Exploring the Use of SVG in React: A Comprehensive Tutorial
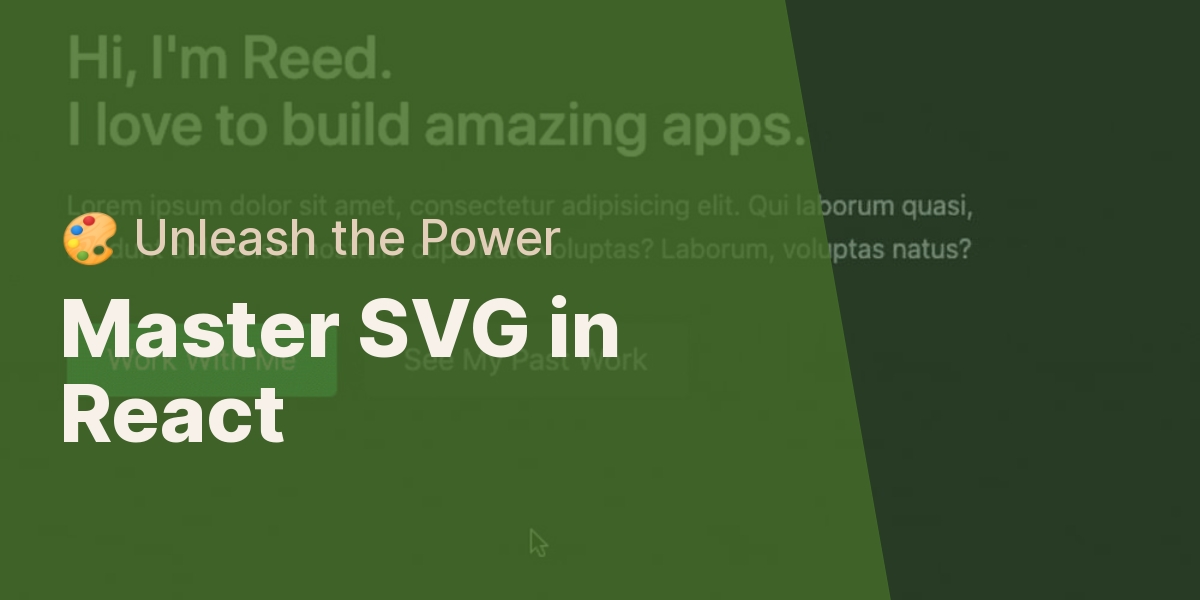
As we delve into the world of SVG in React, it's crucial to first understand what SVG is and why it's so important. SVG, or Scalable Vector Graphics, is an XML-based vector image format for two-dimensional graphics. It's a powerful tool that allows for the creation and manipulation of dynamic, interactive graphics on the web. But why is understanding SVG so essential?
Consider this: Have you ever noticed how some images on the web scale perfectly, regardless of the size of the screen they're viewed on? That's the magic of SVG at work. Unlike raster images, which can become pixelated when enlarged, SVG images maintain their quality at any size. This makes them ideal for responsive web design, a must in today's multi-device world.
When it comes to using SVG with React, the benefits are even more pronounced. React, known for its efficiency and flexibility, pairs perfectly with the scalability of SVG. This combination allows developers to create highly interactive and responsive web applications. Imagine being able to control every aspect of an image, right down to individual pixels, all within your React application.
So, whether you're a seasoned developer looking to expand your toolkit, or a beginner eager to learn, understanding SVG in React is a valuable skill. In this SVG in React tutorial, we'll guide you through the basics, provide a step-by-step implementation guide, and explore common challenges and solutions. By the end, you'll be well on your way to mastering SVG in React.
Ready to dive in? Let's start by exploring the basics of using SVG with React.
Embarking on our journey into the realm of SVG in React, let's first establish the basics. SVG, or Scalable Vector Graphics, is a powerful tool that allows developers to create dynamic, interactive graphics. When paired with React, a JavaScript library known for its efficiency and flexibility, the possibilities are truly endless. But how exactly do we go about using SVG with React?
Firstly, it's important to note that SVG files can be used in React in two primary ways: as an inline SVG or as an SVG file. Inline SVG involves embedding the SVG code directly into your React component. This method provides a high level of control, allowing you to manipulate the SVG elements dynamically using React state and props. On the other hand, using SVG as a file involves importing the SVG file into your component and using it as a standard image source.
Imagine this: You're building a responsive web application. You want to create a dynamic, interactive graphic that scales perfectly, regardless of the size of the screen it's viewed on. With SVG and React, this isn't just possible - it's simple. By manipulating SVG elements within your React application, you can control every aspect of an image, right down to individual pixels.
Exciting, isn't it? But it's not just about the thrill of creating. It's about the practicality and efficiency that using SVG with React brings to your development process. This combination allows you to create highly interactive, scalable, and responsive web applications, making it a valuable skill for any developer.
As we delve deeper into this React SVG tutorial, we'll provide a step-by-step guide on implementing SVG in a React application, explore common challenges and solutions, and share advanced techniques to enhance your React SVG experience. So, are you ready to unlock the full potential of SVG in React?
Now that we've covered the basics, let's dive into the heart of this React SVG tutorial: implementing SVG in a React application. This step-by-step guide will help you understand how to effectively use SVG with React, making your web applications more dynamic and responsive.
First, let's consider using SVG as an inline code in your React component. This is as simple as copying your SVG code and pasting it directly into your component. For example:
{``}
This code creates a purple circle. You can manipulate this SVG element using React state and props, giving you the power to control every aspect of the image.
But what if you want to use SVG as a file? This is where the import statement comes into play. You can import your SVG file into your React component and use it as a standard image source. For instance:
{`import Logo from './logo.svg';`}
Then, you can use it in your component like this:
{`
`}
Remember, the alt attribute provides alternative information for an image if a user cannot view it. It's not just good practice - it's essential for accessibility.
By now, you might be wondering: "Which method should I use?" The answer depends on your specific needs. If you need high control over the SVG elements, inline SVG is the way to go. But if you're looking for a simpler, more straightforward approach, using SVG as a file might be more suitable.
As you can see, using SVG with React opens up a world of possibilities. It's not just about creating beautiful graphics - it's about enhancing the user experience, making your web applications more interactive and responsive. So, are you ready to take your React applications to the next level with SVG?
As we delve deeper into this React SVG tutorial, it's important to acknowledge that, like any other technology, using SVG with React comes with its own set of challenges. But don't worry, we're here to help you navigate these hurdles and find effective solutions.
One common issue you might encounter when using SVG in React is the difficulty in managing complex SVGs. If your SVG code has numerous elements and paths, it can become quite cumbersome to handle. The solution? Break it down. Divide your SVG into smaller, manageable components. This not only makes your code cleaner but also enhances its readability and maintainability.
Another challenge is the performance impact of using inline SVGs. While inline SVGs offer high control, they can also increase the size of your JavaScript bundle, leading to slower load times. If you're dealing with large and complex SVGs, consider using them as files instead. This approach can help optimize your application's performance.
Furthermore, you might face issues with SVG scaling. SVGs are vector-based and should ideally scale without losing quality. However, if not implemented correctly, you might find your SVGs distorting or pixelating. To avoid this, ensure you're using the viewBox attribute correctly. This attribute allows you to specify the aspect ratio and coordinate system of your SVG, ensuring it scales smoothly.
Lastly, let's talk about accessibility. As we mentioned earlier, the alt attribute is crucial for accessibility. But when using SVGs, you also need to consider other factors, like adding descriptive titles and using ARIA attributes. Remember, creating an inclusive web experience is not just about meeting standards - it's about ensuring everyone can enjoy your application, regardless of their abilities.
Overcoming these challenges might seem daunting, but with practice and understanding, you'll soon be able to harness the full potential of using SVG with React. So, are you ready to tackle these challenges head-on and elevate your React SVG game?
Now that we've addressed the common challenges and their solutions, it's time to take your skills to the next level with some advanced techniques. This section of our SVG in React tutorial will help you enhance your React SVG experience, making your applications more efficient and visually appealing.
Firstly, let's talk about optimizing your SVGs. SVGs can sometimes be heavy, especially when they contain a lot of details. This can slow down your application and affect the user experience. One way to overcome this is by using SVG optimization tools like SVGO or SVGOMG. These tools can help you reduce the size of your SVG files without compromising their quality, leading to faster load times and smoother animations.
Another advanced technique involves using CSS with your SVGs. Did you know that you can style your SVGs using CSS? This allows you to change the color, size, and other properties of your SVGs dynamically, offering a high level of customization. For instance, you can change the color of an SVG icon when a user hovers over it, enhancing the interactivity of your application.
Furthermore, consider using SVG sprites if you're dealing with multiple SVG icons. An SVG sprite is a collection of SVGs that are combined into a single file. This can reduce the number of HTTP requests, improving your application's performance.
Lastly, don't forget about animation. SVGs can be animated using CSS or JavaScript libraries like GSAP or Anime.js. This can add a touch of creativity and dynamism to your application, making it more engaging for users.
Remember, mastering these advanced techniques requires practice and patience. But once you get the hang of it, you'll be able to create stunning, efficient, and accessible applications using SVG with React. So, are you ready to take your React SVG skills to the next level?
As we continue our journey through this comprehensive SVG in React tutorial, it's time to introduce you to a resource that will prove invaluable in your quest for SVG mastery: NiceSVG. This platform is your ultimate guide to understanding and using SVG files, providing a wealth of knowledge and tools to help you navigate the world of SVG with ease.
Ever wondered how to open, edit, or save SVG files? NiceSVG has you covered. The platform offers detailed guides and tutorials, making it an excellent resource for both beginners and seasoned developers looking to deepen their understanding of SVG in React. With NiceSVG, you'll find answers to your most pressing SVG-related questions, and gain insights that will help you create more efficient and visually appealing applications.
But that's not all. NiceSVG also boasts a vast library of free SVG files, allowing you to experiment and practice with real SVGs. This hands-on experience is invaluable, providing a practical understanding that complements the theoretical knowledge gained from the tutorials. Whether you're implementing SVG in a React application for the first time or troubleshooting a complex issue, NiceSVG's library is a treasure trove of resources.
And let's not forget about the SVG converters. These handy tools, available on NiceSVG, allow you to convert various file formats into SVGs, and vice versa. This means you can easily integrate SVGs into your existing projects, or convert your SVGs for use in other applications. It's just another way that NiceSVG empowers you to get the most out of SVGs in your React applications.
So, are you ready to explore NiceSVG and take your SVG skills to new heights? Remember, mastering SVG with React is a journey, and NiceSVG is here to guide you every step of the way.
As we draw this comprehensive SVG in React tutorial to a close, it's essential to reflect on the journey we've embarked on together. We've explored the importance of SVG, delved into its basics, and even walked through a step-by-step guide on implementing SVG in a React application. We've tackled common challenges and discovered advanced techniques to enhance your React SVG experience. And, of course, we've introduced you to the invaluable resource that is NiceSVG.
Mastering the use of SVG with React is no small feat. It requires both theoretical knowledge and practical experience. But remember, you're not alone in this journey. With NiceSVG, you have a wealth of resources at your fingertips, from detailed guides and tutorials to a vast library of free SVG files and converters. This platform is more than just a toolβit's your partner in navigating the complex world of SVG.
So, what's next in your journey to understanding SVG in React? Will you dive into the library of free SVG files, experimenting with different designs and techniques? Or perhaps you'll explore the SVG converters, discovering new ways to integrate SVGs into your projects? Whatever path you choose, remember that every step you take brings you closer to mastering SVG in React.
As we wrap up, let's revisit the key takeaway from this React SVG tutorial: SVG is a powerful tool that can enhance your React applications, and NiceSVG is your ultimate guide to harnessing this power. So, don't hesitate. Dive in, explore, and let NiceSVG guide you on your journey to SVG mastery.
Are you ready to take your SVG skills to new heights? NiceSVG is here to guide you every step of the way. Start exploring today!
Post a comment